2 Creating R packages
2.1 Resources
- R Packages by Hadley Wickham and Jenny Bryan
2.2 Creating your first package
Following a similar (but not identical) path to chapter 2 of R Packages by Hadley Wickham and Jenny Bryan, we will build a small package to work with regular expressions. There already are packages that do this sort of thing, so this is just for demonstration purposes.
2.2.1 Step 1: Create a GitHub repo
This isn’t a requirement for building a package, and it doesn’t have to be the first step. But there are many advantages to using github and doing this step first.
As you create your repo:
2.2.1.1 Give it the same name as your package.
This isn’t required, but makes life simpler.
You may name it whatever you like. If you want to match what is in the example by Hadley and Jenny, name it
regexcite
.Note: package names should consist entirely of letters. (Dots are allowed, but please don’t use them in package names.) Unless there is a good reason to use capitals, all lower case is usually preferred.
There is actually a function in R to help you figure out if you have chosen a good name for your package. See
available::available()
.
2.2.1.2 Choose to add a .gitignore
file and select R as the language.
- This will tell git not to track certain files. But the main reason for this is so there is a file in the repo.
2.2.1.3 Optional stuff
Optionally, add a README file
- We will be creating a different README file in a moment.
Optionally, add a license
- Again, we will see a different way to do this in a moment.
2.2.1.4 Add collaborators
If you are working together with others, you can add them as collaborators to your project. This let’s them make changes to your github repository. Alternatively, you could require people to fork your project and issue pull requests to make changes.
2.2.2 Step 2: Connect R and GitHub
Note: If you are working on your own machine, you will need some things that may or may not be installed.
- Xcode Tools (mac) or RTools (PC)
- git
- some R packages (you can install those as we go along)
Assuming those are set up and ready to go, or you are working on https://rstudio.calvin.edu, you should be ready to create an R project for your package.
Set up your SSH key (if you haven’t already done it).
You will need to set up SSH authentication with github if you have not already done this. This is used instead of giving your password to provide access to github.
You can find instructions at GitHub, but there is also an R package that will assist you:
library(credentials) ssh_key_info()
If you don’t have a key set up, you will be prompetd to create one. Say “Yes.”
Copy your key (but not any quotation marks) and paste it as a new key in your GitHub profile.
See https://docs.ropensci.org/credentials/ for more details about
credentials
.In GitHub, copy the GitHub SSH URL from the Code pill (green).
- It should look something like
git@github.com:rpruim/regexcite.git
- It should look something like
In R, create a new project
- Choose Version Control > Git and fill out this dialog
If you leave the project directory name empty, it will name the directory after your github repo name. That’s usually a good option.
You can choose any path for your “Create project as a subdirectory of” as long as it isn’t already under source control.
You should now see something like this:
2.2.3 Step 3: Tell R you want to create a package
The steps so far would be the same for any R-based project we wanted to work
on with GitHub. Now we want to set things up for creating a package.
The source for an R package is just a bunch of files, some with
specified names and/or locations. You can maintain all this manually,
but it is much easier to let the computer take care of some of the bookkeeping
for you. We will do this using the devtools
package.
devtools is a meta-package
As devtools grew, it got unwieldy to maintain and was split into several
packages. The main task of devtools
now is to load all those smaller
packages. So you may see reference to packages like usethis
, remotes
, etc.
2.2.3.1 Attach the devtools
package:
library(devtools)
## Loading required package: usethis
# check the package version
packageVersion('devtools')
## [1] '2.4.3'
2.2.3.2 Run create_package()
You will need to specify where your package code will be located. Since we just set up a new project for this, we’ll put it at the root of the new project. Assuming you haven’t changed your working directory, you can do the following.
R will warn you that you have a project going here already. That’s OK,
we’re just getting started and this is what we intend. But generally you don’t
want to create a new package inside an existing project, so devtools
is
alerting you to the situation.
> create_package(".")
New project 'regexcite' is nested inside an existing project './', which is rarely a good idea.
If this is unexpected, the here package has a function, `here::dr_here()` that reveals why './' is regarded as a project.
Do you want to create anyway?
1: No way
2: Negative
3: Definitely
Selection: 3
Setting active project to '/home/rpruim/github/packages/regexcite'
Creating 'R/'
Writing 'DESCRIPTION'
Package: regexcite
Title: What the Package Does (One Line, Title Case)
Version: 0.0.0.9000
Authors@R (parsed):
* First Last <first.last@example.com> [aut, cre] (YOUR-ORCID-ID)
Description: What the package does (one paragraph).
License: `use_mit_license()`, `use_gpl3_license()` or friends to
pick a license
Encoding: UTF-8
Roxygen: list(markdown = TRUE)
RoxygenNote: 7.1.2
Writing 'NAMESPACE'
Overwrite pre-existing file 'regexcite.Rproj'?
1: I agree
2: Negative
3: Not now
1
Your project should close and reopen and magically some new things appear:
.Rbuildignore lists files that we need to have around but that should not be included when building the R package from source. More in 4.3.1.
.Rproj.user, if you have it, is a directory used internally by RStudio.
.gitignore ignores some standard, behind-the-scenes files created by R and RStudio.
DESCRIPTION provides metadata about your package. We will edit this shortly.
NAMESPACE declares the functions your package exports for external use and the external functions your package imports from other packages. At this point, it is empty, except for a comment declaring that this is a file we will not edit by hand.
The R/ directory is the will soon contain .R files with function definitions.
regexcite.Rproj is the file that makes this directory an RStudio Project. This file stores various settings for your project. You were prompted to overwrite this so that
devtools
could store some information here about your package.
2.2.3.3 Commit your work
It is a good idea to commit your work to git frequently. Let’s do that now. You can do this using command line git in the Terminal tab, or you can use RStudio’s git integration tab:
Notice that blue M. That indicates a file that has been modified. We had
let GitHub create this file for us, and devtools
added some more things to it.
The files with the question marks are not currently part of the repository. We should add them all. (Possible exception: the .Rproj file; there are different opinions about whether this belongs in the repo. I usually do not put it in the repo so that different collaborators can have different RStudio settings for the project.)
Now click commit and add your commit message – perhaps something like “initialize package with create_package().”
If you don’t commit your .Rproj file, git will continue to nag you about it. This is what .gitignore is for. Edit that file so that this file is included and git will know that you want it to be ignored by git.
You should then commit that change as well.
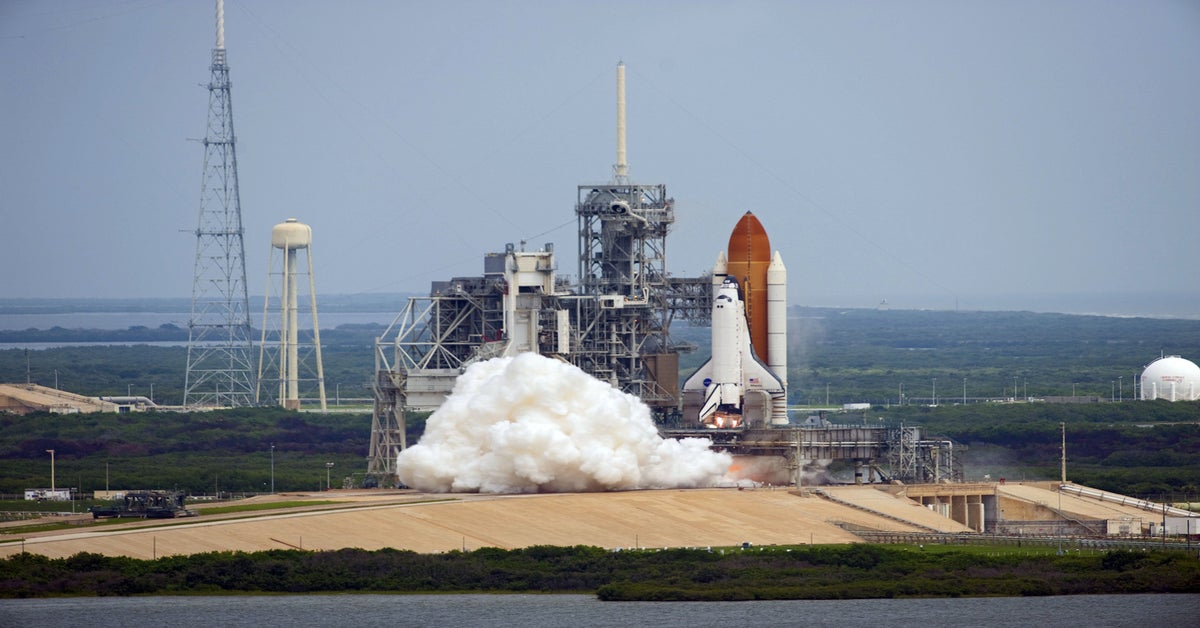
Figure 2.1: Houston, we have ignition!
We have ignition, but we aren’t really going anywhere yet. It’s time to add some functionality to the package.
2.2.4 Make the package do something
Now we are ready to join the walk through from Hadley and Jenny at section 2.6.
A few notes:
The walk-through emphasizes R functions but many of the these things can also be done from the Build tab.
So investigate that as well. You will see that if you use the Build tab, the same commands are executed in the console, but sometimes it is faster/easier to just click rather than type the command (and you don’t have to remember the exact name of the R function).
Don’t do the use_github() section.
If you read that section, you will see that there are multiple ways to connect R and github for package development and the recommendation is to start at GitHub, like we have done. So you are ahead of the game here.